Introduction to web scraping
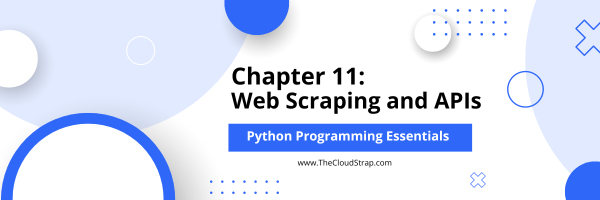
Web scraping and working with APIs are two common ways to retrieve data from the internet in Python.
Web Scraping
This involves programmatically going over a webpage and extracting the information you want. One commonly used library for web scraping in Python is BeautifulSoup. Here’s a simple example:
from bs4 import BeautifulSoup import requests # Make a request to the website r = requests.get('http://www.example.com') r.content # Use the 'html.parser' to parse the page soup = BeautifulSoup(r.content, 'html.parser') # Find the first 'h1' tag and print its contents h1_tag = soup.find('h1') print(h1_tag.text)
Keep in mind that web scraping should be done in accordance with the website’s robots.txt file and terms of service.
APIs
Many websites offer APIs (Application Programming Interfaces) that return data in a structured format, typically JSON, which is much easier to work with than HTML. Here’s a simple example of calling an API using the requests library:
import requests import json # Make a request to the API r = requests.get('http://api.example.com/data') r.content # Parse the JSON data data = json.loads(r.content) # Print the data print(data)
This is a very simple example. Real-world usage would involve more complex URLs, possibly with query parameters, and more complex data processing.
APIs often require authentication, so you might have to provide an API key (usually in the headers) when making your request. They also often have usage limits, so be sure to understand the API’s usage policies before making a large number of requests.
Parsing HTML with Beautiful Soup
Beautiful Soup is a Python library that is used for web scraping purposes to pull the data out of HTML and XML files. Here’s an example of how you could use Beautiful Soup to scrape data from a hypothetical automotive website that lists car prices:
from bs4 import BeautifulSoup import requests # URL of the webpage you want to access url = 'http://www.example-automotive.com' # Send a GET request to the webpage response = requests.get(url) # If the GET request is successful, the status code will be 200 if response.status_code == 200: # Get the content of the response webpage_content = response.content # Create a BeautifulSoup object and specify the parser soup = BeautifulSoup(webpage_content, 'html.parser') # Assume that each car is listed in a div with class 'car-item', and details are in 'car-name' and 'car-price' classes car_divs = soup.find_all('div', class_='car-item') # Loop through each car div and get the car details for car_div in car_divs: car_name = car_div.find('div', class_='car-name').text car_price = car_div.find('div', class_='car-price').text print(f'{car_name}: {car_price}') else: print(f'Failed to retrieve webpage with status code: {response.status_code}')
In the example above, we’re scraping data from a website that lists car prices. For each car, we find the name and price and print it out.
Remember to replace ‘http://www.example-automotive.com’ with the actual URL you want to scrape, and replace the class names with the actual classes used by the website. You’ll have to inspect the HTML of the webpage to find these.
Also, note that this script might not work if the website uses JavaScript to load data or if it has measures in place to prevent web scraping. Always make sure that your web scraping respects the website’s robots.txt file and terms of service.
Making HTTP requests
HTTP requests are a fundamental part of communicating with web services, APIs, and even performing web scraping. Python has a powerful library called requests that simplifies the process of making HTTP requests.
Here’s how you can make various types of HTTP requests using the requests library:
GET Request
This is the most common type of HTTP request. It’s used to retrieve data from a server.
import requests response = requests.get('https://www.example.com') # Print out the response text print(response.text)
POST Request
This type of request is often used to send data to a server.
import requests data = {'key1': 'value1', 'key2': 'value2'} response = requests.post('https://www.example.com', data=data) # Print out the response text print(response.text)
PUT Request
This type of request is used to update a resource on the server.
import requests data = {'key1': 'value1', 'key2': 'value2'} response = requests.put('https://www.example.com', data=data) # Print out the response text print(response.text)
DELETE Request
This type of request is used to delete a resource on the server.
import requests response = requests.delete('https://www.example.com') # Print out the response text print(response.text)
Remember to replace ‘https://www.example.com’ with the URL you want to send a request to, and replace the data dictionaries with the data you want to send.
Each of these methods returns a Response object, which contains the server’s response to your request. You can get the status code of the response with response.status_code, the headers with response.headers, and the response body with response.text or response.content. If the response is JSON, you can use response.json() to automatically parse it into a Python dictionary.
Working with APIs (JSON, RESTful)
Working with APIs involves making HTTP requests and handling HTTP responses. In most cases, data is sent and received in JSON format. The requests library in Python makes this process straightforward.
Here is a simple example of how you might interact with a RESTful API to manage vehicles in a hypothetical automotive software system. Let’s suppose there is an API available at “http://www.automotiveapi.com” for this purpose.
import requests import json # The base URL of the API api_url = 'http://www.automotiveapi.com' # Headers for the API headers = { 'Content-Type': 'application/json' } # The data for the new vehicle new_vehicle = { 'brand': 'Toyota', 'model': 'Camry', 'year': 2023, 'price': 25000 } # POST a new vehicle response = requests.post(api_url + '/vehicles', headers=headers, data=json.dumps(new_vehicle)) # Check if the POST request was successful if response.status_code == 201: print('POST successful. The new vehicle was added.') print('Response:', response.json()) else: print('Failed to POST the new vehicle. Status code:', response.status_code) # GET the list of vehicles response = requests.get(api_url + '/vehicles', headers=headers) # Check if the GET request was successful if response.status_code == 200: print('GET successful. List of vehicles:') vehicles = response.json() for vehicle in vehicles: print(vehicle) else: print('Failed to GET the vehicles. Status code:', response.status_code)
In this script, we are making two types of requests: POST to add a new vehicle to the system, and GET to retrieve the list of vehicles. We’re also checking the status code of the response to see if the request was successful.
Remember to replace the URL, headers, and data with the actual values you need to use for your API. Also, in a real-world scenario, you might need to include authentication in your requests (such as an API key), and the API might impose certain rate limits.
This post was published by Admin.
Email: admin@TheCloudStrap.Com