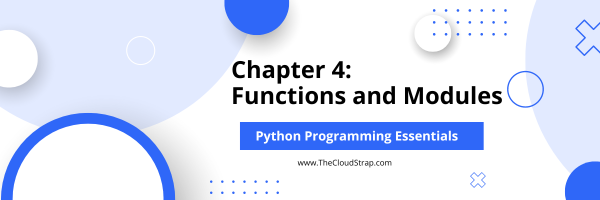
Python is a flexible, undeniable-level programming language utilized across numerous areas including programming improvement, information examination, man-made brainpower, and then some. Its simplicity and clarity make it an attractive choice for developing and testing software, including in the aerospace industry.
Two powerful features of Python are functions and modules. Functions allow you to encapsulate blocks of code that can be reused throughout a program, enhancing readability and reducing repetition. Modules, on the other hand, allow you to organize related functions, variables, and classes into separate files, promoting code reusability and maintainability.
In this blog post, we will explore functions and modules in Python.
Defining and calling functions
In Python, defining a function allows you to group a set of related statements that perform a specific task. This makes it possible to use this set of statements multiple times within a program without re-writing the same code over and over.
As part of the software testing process, let’s say you are an automotive software engineer and have to check a number of subsystems in a vehicle. Here is how you can define and call functions to perform these checks:
First, define the functions:
# Function to perform a brake system check def check_brake_system(): # Code to perform brake system check goes here print("Brake system check complete!") # Function to perform an engine control system check def check_engine_control_system(): # Code to perform engine control system check goes here print("Engine control system check complete!") # Function to perform a transmission control system check def check_transmission_control_system(): # Code to perform transmission control system check goes here print("Transmission control system check complete!")
Each function is defined using the def keyword, followed by the function name and a pair of parentheses. The code block within each function is indented under the function definition and executes when the function is called.
Now, you can call these functions in your software testing process:
# Call the function to perform a brake system check check_brake_system() # Call the function to perform an engine control system check check_engine_control_system() # Call the function to perform a transmission control system check check_transmission_control_system()
When you run the program, it will execute each function call, running the code block defined within each function. The output would be:
In reality, the function bodies would contain code that interacts with the respective subsystems to perform the checks and then perhaps returns a boolean value indicating whether the check passed or failed. However, for simplicity, these details are abstracted here.
By using functions, you can simplify your automotive software testing process, increase the readability of your code, and make it easier to maintain and update your testing process as required.
Function parameters and arguments
In Python, functions can accept parameters, which are values that you can pass into a function when you call it. A function can take any number of parameters. The values you actually pass to the function when calling it are known as arguments.
Here’s how you can use parameters and arguments in functions, with a detailed example from automotive software testing.
Consider you’re writing a program to test the Engine Control Unit (ECU) software in a vehicle. You might define a function that takes two parameters – rpm (revolutions per minute) and load (engine load) and then checks whether these values are within an acceptable range.
Here’s how you could define this function:
def check_ecu_parameters(rpm, load): # Set the acceptable range for rpm and load acceptable_rpm_range = range(800, 6000) # from idle rpm to redline acceptable_load_range = range(0, 101) # expressed as a percentage if rpm not in acceptable_rpm_range: print("RPM out of range!") return False if load not in acceptable_load_range: print("Load out of range!") return False print("ECU parameters are within the acceptable range.") return True
In this function, rpm and load are the parameters. The function checks if these parameters are within the defined ranges, and returns True if they are, and False otherwise.
Now, you can call this function with different arguments to test the ECU:
In these function calls, 850, 30, 7000, 30, and 2000, 120 are the arguments passed to the check_ecu_parameters function.
By using parameters and arguments, you can create flexible functions that perform different operations based on the input they receive, making your code more modular, readable, and reusable.
Return values and scope
In Python, functions can return values using the return statement. These values can then be used elsewhere in your program. The part of a program where a variable is accessible is called its scope. Variables defined inside a function are said to have local scope and can’t be accessed outside that function. Variables defined outside all functions have global scope and can be accessed anywhere in the program.
Here’s a detailed example from automotive software testing to illustrate these concepts:
Consider you’re testing an Engine Control Unit (ECU) software. You define a function check_engine_parameters to validate engine parameters and then use the return value from this function to decide if the engine is functioning correctly.
# Define the function def check_engine_parameters(rpm, load): # Define the acceptable ranges for rpm and load acceptable_rpm_range = range(800, 6000) # from idle rpm to redline acceptable_load_range = range(0, 101) # expressed as a percentage # Validate the parameters and return a status if rpm not in acceptable_rpm_range: return False elif load not in acceptable_load_range: return False else: return True # Call the function and capture its return value engine_status = check_engine_parameters(850, 30) # Use the return value in an if statement if engine_status: print("Engine is functioning correctly.") else: print("Engine parameters out of range!")
In this example, the check_engine_parameters function returns True if the rpm and load parameters are within their acceptable ranges, and False otherwise. The return statement is used to specify this return value.
The variables acceptable_rpm_range and acceptable_load_range are local to the check_engine_parameters function – they are not accessible outside this function. On the other hand, engine_status is a global variable and can be accessed anywhere in the program.
This demonstrates how you can use return values and understand variable scope to structure your code effectively, especially when testing complex systems like automotive software.
Modules and importing
In Python, a module is a file consisting of Python code. A module can define functions, classes, and variables for use in other Python scripts. This concept helps in organizing and reusing code, which is vital in large projects, such as automotive software testing.
To use a Python module in another script or program, you have to import it using the import statement.
Let’s consider a scenario where you’re testing the software of an advanced Driver Assistance System (ADAS) in a car. You might have separate modules for each subsystem, such as lane_detection.py, adaptive_cruise_control.py, and automatic_emergency_braking.py. Each of these modules contains functions related to testing its respective system.
Here’s an example of what one of these modules might look like:
# lane_detection.py def check_lane_detection(): # Code to perform a lane detection test goes here print("Lane detection test complete!")
You can import the lane_detection module and use the check_lane_detection function in your main test script as follows:
# main_test_script.py # Import the lane_detection module import lane_detection # Run the lane detection test lane_detection.check_lane_detection() # Output: "Lane detection test complete!"
In this example, the import statement imports the lane_detection module, which allows you to use the check_lane_detection function in your main test script.
Python also provides the ‘from … import … ‘ statement to import specific functions from a module, which can make your code cleaner if you only need to use a few functions from a module:
# main_test_script.py # Import the check_lane_detection function from the lane_detection module from lane_detection import check_lane_detection # Run the lane detection test check_lane_detection() # Output: "Lane detection test complete!"
In this case, the check_lane_detection function is imported directly, so you don’t need to use the module name to call it.
Python’s support for modules and easy import mechanism helps in creating well-organized, reusable, and maintainable code – a crucial aspect when working on complex tasks like automotive software testing.
Standard library and third-party modules
Python provides a rich set of standard library modules that can be readily imported into your Python scripts to perform a wide range of tasks. These include modules for file I/O, system calls, dates and times, regular expressions, and much more.
On top of the standard library, there’s also an extensive ecosystem of third-party Python modules, which are distributed separately from Python and maintained by individual developers or teams. These modules often provide more specialized or advanced functionality.
Here are examples of using both standard library and third-party modules.
Standard Library Modules
One frequently used module in the Python standard library is datetime, which provides classes for manipulating dates and times.
import datetime # Get the current date and time now = datetime.datetime.now() # Print the current date and time print(now) # Output: e.g. "2023-06-01 12:45:28.292192" # Create a date object date = datetime.date(2023, 6, 1) # Print the date print(date) # Output: "2023-06-01"
In this example, the datetime module is imported, and then its datetime and date classes are used to work with dates and times.
Third-Party Modules
An example of a widely used third-party Python module is requested, which allows you to send HTTP requests easily.
import requests # Make a GET request to an API response = requests.get("https://api.github.com") # Print the status code print(response.status_code) # Output: 200 # Print the response content print(response.json())
In this example, the requests module is imported, and its get function is used to send a GET request to the GitHub API. The response status code and content are then printed.
Note that third-party modules like requests need to be installed separately before they can be imported, usually with a package manager like pip. For example, to install requests, you would run pip install requests in your command line.
By making use of both standard library and third-party modules, you can greatly expand the capabilities of Python and avoid reinventing the wheel, focusing instead on the unique parts of your application or script.
This post was published by Admin.
Email: admin@TheCloudStrap.Com