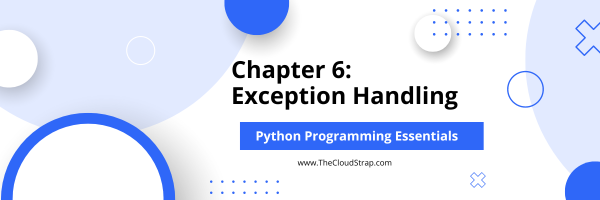
The world of software testing, particularly in specialized fields such as automotive software, is filled with opportunities for things to go awry. Unforeseen circumstances can lead to erroneous behavior or outright failures in the software we are trying to test. In Python, we handle these unforeseen events through a mechanism known as exception handling. Let’s dig deeper into this topic.
What is Exception Handling?
Exception handling in Python involves the use of try, except, finally, and raise blocks to “catch” and respond to errors (exceptions) during the execution of your program. This allows your program to continue running even when an error occurs, or to provide more informative error messages to the user.
The Need for Exception Handling in Software
When testing automotive software, we may encounter numerous unpredictable situations. For instance, a test case may fail, or a piece of equipment connected to your system via a network may become unresponsive. Without exception handling, these scenarios could cause your entire testing suite to crash, wasting time and resources.
With exception handling, we can catch these errors and respond accordingly—perhaps by logging the error, retrying the test, skipping over the problematic test case, or notifying the user.
Python Exception Handling: An Example in Automotive Software Testing
Consider a simple Python function that simulates a test on an automotive component:
def run_test(test_case, component): if component.is_functional(): result = component.run_test(test_case) else: raise Exception(f"Component {component.name} is not functional.") return result
If the component is not functional, the function raises an exception and the testing process stops. But we don’t want our entire testing suite to stop just because one test fails. This is where exception handling comes in.
for test_case in test_cases: for component in components: try: result = run_test(test_case, component) print(f"Test {test_case.id} passed with result: {result}") except Exception as e: print(f"An error occurred: {e}")
In this code, we use a try block to attempt to run the test. If an error occurs while running the test—because the component is not functional, for example—the code in the except block is executed, and a message is printed to the screen.
In automotive software testing, we often need to communicate with external devices or components that could raise exceptions due to various reasons such as network issues, timeout errors, or data transmission problems. Exception handling via a try and except can handle such issues gracefully and allow your test suite to continue.
Let’s take an example where we are communicating with a car’s onboard diagnostics system. We have a function that requests diagnostic trouble codes (DTCs) from the vehicle.
import random class DiagnosticTool: def request_dtc(self): """Request DTCs from the vehicle. This is a simulated function for the example.""" # There is a 10% chance the request will fail if random.random() < 0.1: raise ConnectionError("Failed to communicate with vehicle.") # Return some mock DTCs return ["P0442", "P0171"] # Now we use the above function in our test diagnostic_tool = DiagnosticTool() for i in range(10): try: dtcs = diagnostic_tool.request_dtc() print(f"Received DTCs: {dtcs}") except ConnectionError as e: print(f"Attempt {i+1}: Could not fetch DTCs due to error: {e}")
In this code, diagnostic_tool.request_dtc() has a 10% chance to raise a ConnectionError simulating a failure in communicating with the vehicle. The try/except block allows the test to catch this exception and print an error message, instead of crashing the entire test suite.
This way, even if we encounter an error, we can inform the user about the error and continue with the remaining tests, thus improving the robustness of the test suite.
Raising and catching custom exceptions
In Python, you can define your own exceptions by creating a new class. This class should derive from the built-in Exception class. You can then raise your custom exceptions with the raise keyword.
In the context of automotive software testing, you might define custom exceptions to handle specific error conditions in your application. For example, you might define an ECUConnectionError for errors related to connecting to an ECU, and an ECUDataReadError for errors related to reading data from an ECU.
Here’s how you might define these exceptions:
class ECUConnectionError(Exception): """Exception raised for errors in the ECU connection.""" pass class ECUDataReadError(Exception): """Exception raised for errors in reading data from ECU.""" pass
These exceptions can then be raised and caught in your application:
import random class ECU: """Represents an Electronic Control Unit in a car.""" def connect(self): """Simulate connecting to the ECU.""" # Simulate a chance of failure if random.random() < 0.1: raise ECUConnectionError("Failed to connect to ECU.") def disconnect(self): """Simulate disconnecting from the ECU.""" print("Disconnected from ECU.") def read_data(self): """Simulate reading data from the ECU.""" # Simulate a chance of failure if random.random() < 0.1: raise ECUDataReadError("Error reading data from ECU.") return {"RPM": 3000, "Speed": 60} def test_ecu(ecu): """Run a test on the given ECU.""" try: print("Attempting to connect to ECU...") ecu.connect() print("Connected to ECU.") except ECUConnectionError as e: print(e) else: try: print("Reading data from ECU...") data = ecu.read_data() print(f"Received data from ECU: {data}") except ECUDataReadError as e: print(e) finally: print("Cleaning up...") ecu.disconnect() # Create an ECU instance and run the test ecu = ECU() for _ in range(5): print("Running test...\n") test_ecu(ecu) print("\n")
In this updated code, instead of raising generic ConnectionError and RuntimeError exceptions, we now raise our custom ECUConnectionError and ECUDataReadError exceptions. These custom exceptions can give you more control over your error handling and make your code easier to understand.
Cleaning up finally
The finally block allows you to specify code that will be executed no matter what—whether an exception is raised or not. This is useful for cleanup tasks, such as closing a file or a network connection. In the context of automotive testing, you might use a finally block to ensure that the component is reset to its initial state after each test:
for test_case in test_cases: for component in components: try: result = run_test(test_case, component) print(f"Test {test_case.id} passed with result: {result}") except Exception as e: print(f"An error occurred: {e}") finally: component.reset()
Now, let’s dive into a more complex scenario that uses try, except, and finally in the context of automotive software testing. In this example, we’ll test communication with an Electronic Control Unit (ECU) in a car:
import random class ECU: """Represents an Electronic Control Unit in a car.""" def connect(self): """Simulate connecting to the ECU.""" # Simulate a chance of failure if random.random() < 0.1: raise ConnectionError("Failed to connect to ECU.") def disconnect(self): """Simulate disconnecting from the ECU.""" print("Disconnected from ECU.") def read_data(self): """Simulate reading data from the ECU.""" # Simulate a chance of failure if random.random() < 0.1: raise RuntimeError("Error reading data from ECU.") return {"RPM": 3000, "Speed": 60} def test_ecu(ecu): """Run a test on the given ECU.""" try: print("Attempting to connect to ECU...") ecu.connect() print("Connected to ECU.") except ConnectionError as e: print(e) else: try: print("Reading data from ECU...") data = ecu.read_data() print(f"Received data from ECU: {data}") except RuntimeError as e: print(e) finally: print("Cleaning up...") ecu.disconnect() # Create an ECU instance and run the test ecu = ECU() for _ in range(5): print("Running test...\n") test_ecu(ecu) print("\n")
In this example, we have an ECU class that simulates the behavior of an electronic control unit in a car. The ECU has three methods: connect(), disconnect(), and read_data(). Both connect() and read_data() have a 10% chance to raise an error.
The test_ecu() function is designed to test communication with the ECU. It uses a try/except/else/finally block structure:
The try block attempts to connect to the ECU. If a ConnectionError is raised, the except block is executed, and an error message is printed.
If no ConnectionError is raised, the else block is executed. This block contains another try/except block that attempts to read data from the ECU. If a RuntimeError is raised, the except block is executed, and an error message is printed.
The finally block is executed no matter what. It disconnects from the ECU, ensuring that every connection is properly closed, even if an error occurs.
Conclusion
Exception handling is a powerful tool for creating robust, reliable software—especially in complex, unpredictable environments like automotive software testing. By understanding and using Python’s try, except, finally, and raise blocks, you can ensure that your testing suite continues to function under adverse conditions and that your tests provide useful, actionable information even when things go wrong.
This post was published by Admin.
Email: admin@TheCloudStrap.Com