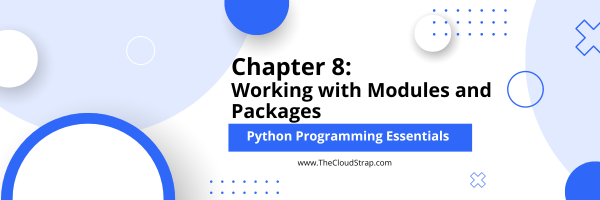
Python modules and packages are ways to organize large amounts of code. In essence, a module is a single Python file, while a package is a directory of Python modules containing an additional __init__.py file.
To illustrate, consider this directory structure:
In this example, my_package is a package that contains two modules: module1 and module2.
Let’s assume that module1.py contains the following code:
# module1.py def say_hello(name): print(f"Hello, {name}!")
And module2.py contains:
# module2.py def say_goodbye(name): print(f"Goodbye, {name}!")
You could use the functions from these modules in main.py like this:
# main.py from my_package.module1 import say_hello from my_package.module2 import say_goodbye say_hello('Alice') # Hello, Alice! say_goodbye('Alice') # Goodbye, Alice!
This import statement is saying, “from the package my_package, import the module module1, and from module1 import the function say_hello.”
You can also import a whole module or a whole package. For example:
# Import the whole module import my_package.module1 my_package.module1.say_hello('Alice')
# Import the whole package import my_package my_package.module1.say_hello('Alice') my_package.module2.say_goodbye('Alice')
The __init__.py file serves to initialize the package. It’s executed when the package is imported, and it can contain any Python code. However, it’s often left empty or is used to specify what gets imported when you import * from the package.
In short, modules and packages are an important part of Python because they allow you to organize and reuse your code in a logical way.
Creating and organizing modules
Let’s consider a complex project structure for a hypothetical automotive software system. This will involve several modules and packages.
Consider the following directory structure:
In car.py, we define a general Car class:
# car.py class Car: def __init__(self, brand, model, year): self.brand = brand self.model = model self.year = year def display_info(self): return f'{self.brand} {self.model} ({self.year})'
In electric_car.py and gas_car.py, we define specific types of cars:
# electric_car.py from .car import Car class ElectricCar(Car): def __init__(self, brand, model, year, battery_capacity): super().__init__(brand, model, year) self.battery_capacity = battery_capacity
# gas_car.py from .car import Car class GasCar(Car): def __init__(self, brand, model, year, fuel_capacity): super().__init__(brand, model, year) self.fuel_capacity = fuel_capacity
In maintenance.py, we define a general Maintenance class:
# maintenance.py class Maintenance: def perform_maintenance(self, car): pass
In battery_maintenance.py and engine_maintenance.py, we define specific types of maintenance:
# battery_maintenance.py from .maintenance import Maintenance class BatteryMaintenance(Maintenance): def perform_maintenance(self, car): print(f'Performing battery maintenance on {car.brand} {car.model}')
# engine_maintenance.py from .maintenance import Maintenance class EngineMaintenance(Maintenance): def perform_maintenance(self, car): print(f'Performing engine maintenance on {car.brand} {car.model}')
Finally, in main.py, we can import and use all of these classes:
# main.py from car.car import Car from car.electric_car import ElectricCar from car.gas_car import GasCar from maintenance.battery_maintenance import BatteryMaintenance from maintenance.engine_maintenance import EngineMaintenance car1 = ElectricCar('Tesla', 'Model S', 2023, 100) car2 = GasCar('Ford', 'Mustang', 2023, 60) battery_maintenance = BatteryMaintenance() engine_maintenance = EngineMaintenance() battery_maintenance.perform_maintenance(car1) # Performing battery maintenance on Tesla Model S engine_maintenance.perform_maintenance(car2) # Performing engine maintenance on Ford Mustang
Note that in the import statements, we’re using dot notation to specify the path to the modules. The .py extension is not included in the import statement.
This is a basic example, but it shows how you can use modules and packages to structure a complex Python project. In a real-world application, you’d have many more modules and classes, and your classes would have more complex behavior.
Packages and Sub-Packages
In Python, packages can contain sub-packages, which are just directories inside the package directory that contain their own Python modules and possibly their own sub-packages.
Consider the following directory structure:
In this structure, car is a package that contains two sub-packages: electric and gas. Each sub-package contains a module (electric_car.py and gas_car.py) that defines a specific type of car.
Here’s what the car.py, electric_car.py, and gas_car.py modules might look like:
# car.py class Car: def __init__(self, brand, model, year): self.brand = brand self.model = model self.year = year def display_info(self): return f'{self.brand} {self.model} ({self.year})'
# electric_car.py from ..car import Car class ElectricCar(Car): def __init__(self, brand, model, year, battery_capacity): super().__init__(brand, model, year) self.battery_capacity = battery_capacity
# gas_car.py from ..car import Car class GasCar(Car): def __init__(self, brand, model, year, fuel_capacity): super().__init__(brand, model, year) self.fuel_capacity = fuel_capacity
You can see in the ElectricCar and GasCar classes that we’re using the .. syntax in the import statement. This is a relative import, and it means going up one level in the package hierarchy.
Finally, in main.py, you could use these classes like so:
# main.py from car.car import Car from car.electric.electric_car import ElectricCar from car.gas.gas_car import GasCar car1 = ElectricCar('Tesla', 'Model S', 2023, 100) car2 = GasCar('Ford', 'Mustang', 2023, 60) print(car1.display_info()) # Tesla Model S (2023) print(car2.display_info()) # Ford Mustang (2023)
In the import statements, we’re using dot notation to specify the path to the modules, including the sub-packages. The .py extension is not included in the import statement.
This example shows how packages and sub-packages can be used to organize complex Python projects. In a real-world application, you’d have many more sub-packages and modules, and your classes would have more complex behavior.
Importing modules and packages
Let’s extend our previous automotive software example to illustrate importing modules, packages, and sub-packages in Python.
Consider the following directory structure:
Let’s assume that car.py, electric_car.py, and gas_car.py are the same as in the previous example.
In maintenance.py, we define a general Maintenance class:
# maintenance.py class Maintenance: def perform_maintenance(self, car): pass
In battery_maintenance.py and engine_maintenance.py, we define specific types of maintenance:
# battery_maintenance.py from ...car.electric.electric_car import ElectricCar from ..maintenance import Maintenance class BatteryMaintenance(Maintenance): def perform_maintenance(self, car): assert isinstance(car, ElectricCar), "Can only perform battery maintenance on an electric car." print(f'Performing battery maintenance on {car.brand} {car.model}') # engine_maintenance.py from ...car.gas.gas_car import GasCar from ..maintenance import Maintenance class EngineMaintenance(Maintenance): def perform_maintenance(self, car): assert isinstance(car, GasCar), "Can only perform engine maintenance on a gas car." print(f'Performing engine maintenance on {car.brand} {car.model}')
You can see in the BatteryMaintenance and EngineMaintenance classes that we’re using the … syntax in the import statement. This is a relative import, and it means going up two levels in the package hierarchy.
Finally, in main.py, you could use these classes like so:
# main.py from car.electric.electric_car import ElectricCar from car.gas.gas_car import GasCar from maintenance.electric.battery_maintenance import BatteryMaintenance from maintenance.gas.engine_maintenance import EngineMaintenance car1 = ElectricCar('Tesla', 'Model S', 2023, 100) car2 = GasCar('Ford', 'Mustang', 2023, 60) battery_maintenance = BatteryMaintenance() engine_maintenance = EngineMaintenance() battery_maintenance.perform_maintenance(car1) # Performing battery maintenance on Tesla Model S engine_maintenance.perform_maintenance(car2) # Performing engine maintenance on Ford Mustang
In the import statements, we’re using dot notation to specify the path to the modules, including the sub-packages. The .py extension is not included in the import statement.
This example shows how to use and organize complex Python projects by importing modules, packages, and sub-packages. In a real-world application, you’d have many more sub-packages and modules, and your classes would have more complex behavior.
Exploring Python’s standard library
Python’s standard library is a set of modules included with every Python installation. It provides a wide range of functionalities, including mathematical operations, file I/O, system operation, and much more.
Let’s explore some of the parts of Python’s standard library that might be used in an automotive software system.
datetime
The datetime module allows you to work with dates and times. It can be used to track the manufacturing date of cars and the dates of maintenance tasks.
import datetime class Car: def __init__(self, brand, model, year): self.brand = brand self.model = model self.year = year self.manufacture_date = datetime.datetime.now() def age_in_days(self): return (datetime.datetime.now() - self.manufacture_date).days
In this example, each Car instance has a manufacture_date attribute, which is set to the current date and time when the car is created. The age_in_days method returns the age of the car in days.
math
The math module provides mathematical functions. In an automotive context, it might be used to calculate things like the range of a car based on its fuel efficiency and the amount of fuel in its tank.
import math class GasCar(Car): def __init__(self, brand, model, year, fuel_capacity, fuel_efficiency): super().__init__(brand, model, year) self.fuel_capacity = fuel_capacity self.fuel_efficiency = fuel_efficiency # in km per liter def calculate_range(self): return math.floor(self.fuel_capacity * self.fuel_efficiency)
In this example, each GasCar instance has a calculate_range method that calculates the car’s range based on its fuel capacity and fuel efficiency.
os and shutil
The os and shutil modules provide functions for interacting with the operating system. This could be used, for example, to manage files related to different cars or maintenance tasks.
import os import shutil class Maintenance: def __init__(self, maintenance_folder): self.maintenance_folder = maintenance_folder def create_maintenance_record(self, car): record_path = os.path.join(self.maintenance_folder, f'{car.brand}_{car.model}_{car.year}.txt') with open(record_path, 'w') as f: f.write(f'Maintenance performed on {car.brand} {car.model} ({car.year}) on {datetime.datetime.now()}') def archive_maintenance_record(self, car): record_path = os.path.join(self.maintenance_folder, f'{car.brand}_{car.model}_{car.year}.txt') archive_folder = os.path.join(self.maintenance_folder, 'archive') os.makedirs(archive_folder, exist_ok=True) shutil.move(record_path, archive_folder)
In this example, the Maintenance class has methods for creating and archiving maintenance records. These methods use the os and shutil modules to create and move files.
Remember, Python’s standard library is very extensive and includes much more than the few modules shown here. The full documentation is available at https://docs.python.org/3/library/.
Conclusion
Their usage promotes code reusability, a hallmark of effective programming, by providing the means to compartmentalize and reapply code.
On a larger scale, Packages — essentially directories of Python modules — provide an additional layer of organization, effectively forming a hierarchy of modules. This ability to bundle related modules into Packages contributes to the development of larger, more complex applications, while maintaining structure and clarity.
Moreover, Python’s extensive ecosystem of third-party Packages, accessible through tools like pip, further extends the language’s capabilities across a wide range of domains. This rich assortment of Packages, from NumPy and Pandas for data analysis to Django and Flask for web development, empowers Python developers to tackle virtually any programming challenge.
However, the true power of Modules and Packages isn’t just in their utility as organizational tools or code repositories. Their most significant impact is on developer efficiency and productivity.
In conclusion, the ability to effectively use Modules and Packages in Python is a fundamental skill that amplifies a developer’s potential. As such, they are vital constructs for both the novice Python programmer and the seasoned Python developer.
This post was published by Admin.
Email: admin@TheCloudStrap.Com