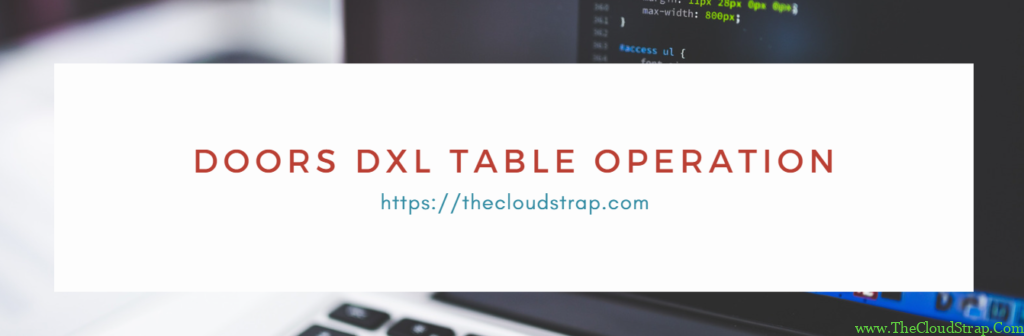
In this article, we will look into DOORS DXL table operations.
In Rational DOORS, a table is an object hierarchy displayed in the form of a table. The table’s top level is referred to as the table header object; for each row, it has a sub-object, called a row object. These row objects, in turn, have sub-objects, which are the table cells.
DOORS tables consist of
- one “table” object for the table
- one “row” object for each row. These row objects are children of the table object
- one “cell” object for each cell in a row. These cell objects are children of their respective row objects.
To view the table cell only, you need to switch off “View -> Show -> Table Cells”.
Create and Insert data into Table
Here are the following functions that can be used to create a table in DXL –
Object table(Module m, int rows, int cols)
Object table(Object o, int rows, int cols)
Object table(before(Object o), int rows, int cols)
Object table(below(Object o), int rows, int cols)
Object table(last(below(Object o)), int rows, int cols)
The first form creates a table of size rows, cols as the first object in a module.
The second form creates a table of size rows, cols at the same level and immediately after object o.
The third form creates a table of size rows, cols at the same level and immediately before the object o.
The fourth form creates a table of size rows, cols as the first child of the object o.
The fifth form creates a table of size rows, cols as the last child of the object o
Now, let’s look at an example code –

// www.TheCloudStrap.Com /************************************************************************ * $FILENAME: tb1.dxl * $DESCRIPTION: Table example in DXL * * NOTICE: Copyright www.TheCloudStrap.Com. All rights reserved. * Software comes without any warranties and guarantees, is provided * as is and is not supported. Use this software at your own risk. * Authors resume no liabilities. * * Contact: admin {at} TheCloudStrap.com ************************************************************************/ // Table example in DXL Module m = current Object t1, t2 Object lastObj = last(m) // Create table t1 = table (before lastObj, 3, 3) t2 = table (m, 5, 5) // insert data into table Object o, r, c for o in document current Module do { if(table(o)) { print "Table object found " identifier (o) "\n" for r in table o do { print "Row found " identifier (r) "\n" for c in row r do { print "Cell found " identifier (c) "\n" c."Object Text" = "sample_data_" lower(identifier (c)) "" } } } } print "Tables created!"
Here is the output –


Here is the syntax for row navigation in the table –
for ro in table(Object o) do { ... }
Here, ro is a row variable of type Object and o is an object of type Object.
Here is the syntax for cell navigation in Table –
for co in row(Object o) do { ... }
Here, co is a cell variable of type Object and o is an object of type Object.
Table Header
A for loop can be used to navigate through the table rows and columns. Here is an example to demonstrate the table navigation. The following example code navigates through the table headers only.
// www.TheCloudStrap.Com /************************************************************************ * $FILENAME: tb2.dxl * $DESCRIPTION: Table example in DXL * * NOTICE: Copyright www.TheCloudStrap.Com. All rights reserved. * Software comes without any warranties and guarantees, is provided * as is and is not supported. Use this software at your own risk. * Authors resume no liabilities. * * Contact: admin {at} TheCloudStrap.com ************************************************************************/ // Table example in DXL Module m = current // Table navigation Object o, r, c // This for loop respects all display set objects and table headers only for o in document current Module do { if(table(o)) { print "Table object found " identifier (o) "\n" for r in table o do { print "Row found " identifier (r) "\n" for c in row r do { //print "Cell found " identifier (c) "\n" print ">> Cell Data = " c."Object Text" "\n" } } } } print "Done!"

Table Row and Cells
In the following example, the table header, row, and cell navigation are demonstrated –
// www.TheCloudStrap.Com /************************************************************************ * $FILENAME: tb3.dxl * $DESCRIPTION: Table example in DXL * * NOTICE: Copyright www.TheCloudStrap.Com. All rights reserved. * Software comes without any warranties and guarantees, is provided * as is and is not supported. Use this software at your own risk. * Authors resume no liabilities. * * Contact: admin {at} TheCloudStrap.com ************************************************************************/ // Table example in DXL Module m = current // Table navigation Object o, r, c // This for loop respects all display set objects including table, row headers and cells for o in all current Module do { if(table(o)) { print "Table object found " identifier (o) "\n" for r in table o do { print "Row found " identifier (r) "\n" for c in row r do { //print "Cell found " identifier (c) "\n" print ">> Cell Data = " c."Object Text" "\n" } } } } print "Done!"

Other Table manipulation functions
Here are the other table manipulation functions that can be useful while writing real-world complex dxl programs –
Object appendColumn(Object tableCell)
Object appendRow(Object tableCell)
Object insertCell(Object tableCell)
Object insertColumn(Object tableCell)
Object insertRow(Object tableCell)
void setCellWidth(Object tableCell, int width)
void setColumnWidth(Object tableCell, int width)
void deleteTable(Object tableHeader)
Conclusion
In this article, we have discussed DOORS DXL Table operations i.e. – how to create a table and handle different ways of navigation using DXL.
This post was published by Admin.
Email: admin@TheCloudStrap.Com