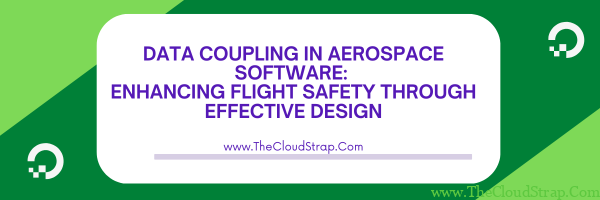
Introduction
A brief definition of data coupling:
Data coupling refers to a scenario in software development where one module directly interacts with another by passing data (like variables or parameters). In essence, it’s the degree to which one module relies on another module’s data. This concept is part of ‘coupling’, a broader term in software engineering that describes the interdependencies between different parts of a software system.
Overview of aerospace software:
Aerospace software is a critical component of aviation systems, from navigation and control systems to onboard communication and monitoring systems. This software is designed to meet extremely high standards of safety and reliability, as any failure can lead to catastrophic results. It’s used in a variety of platforms, including aircraft, spacecraft, satellites, and unmanned aerial vehicles (UAVs).
Importance of data coupling in aerospace software:
Given the critical nature of aerospace software, understanding and managing data coupling is of utmost importance. High levels of data coupling can lead to systems that are difficult to maintain, test, and update. On the other hand, well-managed data coupling can facilitate a modular and robust system, capable of withstanding the demanding environment of aerospace applications. The remainder of this blog post will delve into this subject in detail, exploring the implications, challenges, and management strategies related to data coupling in aerospace software.
Understanding Data Coupling
A detailed explanation of data coupling:
Data coupling is a measure of how much and in what ways one software module (like a function, class, or component) is directly dependent on another through data exchange. This usually happens when one module shares data with another, typically through parameters or variables. Data coupling is considered a low degree of coupling, which is often desirable because it contributes to a software design that is easier to maintain, test, and debug. However, excessive data coupling can still lead to issues if changes in one module require extensive changes in others due to their data dependencies.
Types of data coupling:
There are several forms of data coupling. For example, simple data coupling occurs when one module passes data to another, but the receiving module doesn’t modify that data. Control coupling is a form of data coupling where one module controls the flow of another by passing control information (like a flag). Stamp coupling happens when multiple pieces of data are passed together in a data structure, even if the receiving module only needs a subset of that data. These are just a few examples.
The concept of coupling and its significance in software engineering:
In the broader context, coupling refers to the degree of interdependence between software modules. A high degree of coupling means that changes in one module are likely to affect others, leading to software that is harder to maintain and modify. The goal in software engineering is often to minimize coupling where possible, leading to more modular and robust software. This principle is part of a broader set of design guidelines known as modularity, encapsulation, and information hiding, all aimed at creating maintainable and reliable software systems.
Data Coupling in Aerospace Software
The role of data coupling in aerospace software:
In aerospace software, data coupling plays a crucial role due to the integrated and complex nature of these systems. Each module in an aircraft’s or satellite’s software system can be responsible for a specific function, such as navigation, communication, or environmental control, and these modules often need to share data. The way this data coupling is managed can significantly affect the software’s performance, maintainability, and safety.
Real-world examples of data coupling in aerospace systems:
One common example of data coupling in aerospace software is the interaction between navigation and flight control systems in an aircraft. The navigation system provides data like the aircraft’s current position, altitude, and speed to the flight control system, which uses this data to adjust the aircraft’s control surfaces and maintain the correct flight path. This is a clear case of data coupling, where changes in the navigation system data (like a new destination) would directly affect the operation of the flight control system.
Data Coupling Example-1:
In the context of aerospace software, we can think of an example involving a flight control system and a navigation system. Let’s say we have two modules, one to calculate the altitude and the other to control the engine based on that altitude.
Here’s a simple representation of C programming:
// Module 1: Navigation System typedef struct { double altitude; double longitude; double latitude; } NavigationData; void calculateAltitude(NavigationData* navData) { // Assume this function calculates altitude based on other factors navData->altitude = /* Calculated Altitude */; // Also calculates longitude and latitude navData->longitude = /* Calculated Longitude */; navData->latitude = /* Calculated Latitude */; }
// Module 2: Flight Control System void controlEngine(NavigationData* navData) { if (navData->altitude > 10000.0) { // Code to reduce the engine thrust } else if (navData->altitude < 5000.0) { // Code to increase the engine thrust } else { // Code to maintain the engine thrust } } // Main function demonstrating data coupling int main() { NavigationData navData; calculateAltitude(&navData); controlEngine(&navData); return 0; }
In this example, the Navigation System module calculates the altitude and updates a NavigationData struct. The Flight Control System module then reads the altitude from the NavigationData struct to control the engine. These modules are data coupled because the Flight Control System module directly depends on the data from the Navigation System module.
This is an example of simple data coupling, which is a low level of coupling and generally desirable. However, changes to the NavigationData struct (like renaming or removing the altitude field) could potentially affect the Flight Control System module, demonstrating why it’s important to manage data coupling effectively.
Data Coupling Example-2:
Let’s consider an example of data coupling in the context of aerospace software involving a sensor data processing system and a flight control system. The sensor data processing system receives data from multiple sensors, processes it, and then passes it on to the flight control system to make necessary adjustments.
Here’s a simple representation of C programming:
// Module 1: Sensor Data Processing System typedef struct { double altitude; double airspeed; double angle_of_attack; } SensorData; void processSensorData(SensorData* sensorData) { // Assume this function processes data received from various sensors sensorData->altitude = /* Processed Altitude */; sensorData->airspeed = /* Processed Airspeed */; sensorData->angle_of_attack = /* Processed Angle of Attack */; }
// Module 2: Flight Control System void controlFlightSystem(SensorData* sensorData) { if (sensorData->airspeed < 120.0) { // Code to increase engine thrust } else if (sensorData->airspeed > 180.0) { // Code to decrease engine thrust } else { // Code to maintain engine thrust } if (sensorData->angle_of_attack > 15.0) { // Code to adjust control surfaces for reducing angle of attack } else if (sensorData->angle_of_attack < -15.0) { // Code to adjust control surfaces for increasing angle of attack } } // Main function demonstrating data coupling int main() { SensorData sensorData; processSensorData(&sensorData); controlFlightSystem(&sensorData); return 0; }
In this example, the Sensor Data Processing System module processes data from various sensors and updates a SensorData struct. The Flight Control System module then reads the data from the SensorData struct to adjust the engine thrust and control surfaces accordingly. These modules are data coupled because the Flight Control System module directly depends on the data from the Sensor Data Processing System module.
This is an example of simple data coupling, which is a low level of coupling and generally desirable. However, changes to the SensorData struct (like renaming or removing any field) could potentially affect the Flight Control System module, emphasizing the importance of managing data coupling effectively.
Data Coupling Example-3:
Let’s consider another example involving an engine monitoring system and an engine control system in aerospace software. The engine monitoring system collects data from various sensors in the engine, and the engine control system uses this data to control the engine’s operations.
Here’s a basic representation of C programming:
// Module 1: Engine Monitoring System typedef struct { double temperature; double pressure; double rpm; } EngineData; void collectEngineData(EngineData* engineData) { // Assume this function collects data from various engine sensors engineData->temperature = /* Collected Temperature */; engineData->pressure = /* Collected Pressure */; engineData->rpm = /* Collected RPM */; }
// Module 2: Engine Control System void controlEngine(EngineData* engineData) { if (engineData->temperature > 900.0) { // Code to reduce engine thrust to lower temperature } if (engineData->pressure < 20.0) { // Code to increase fuel flow to increase pressure } if (engineData->rpm > 12000.0) { // Code to reduce fuel flow to lower RPM } } // Main function demonstrating data coupling int main() { EngineData engineData; collectEngineData(&engineData); controlEngine(&engineData); return 0; }
In this example, the Engine Monitoring System module collects data from various engine sensors and updates an EngineData struct. The Engine Control System module then reads the data from the EngineData struct to control the engine’s operations. These modules are data coupled because the Engine Control System module directly depends on the data from the Engine Monitoring System module.
This is an example of simple data coupling, which is a low level of coupling and generally desirable. However, changes to the EngineData struct (like renaming or removing any field) could potentially affect the Engine Control System module, emphasizing the importance of managing data coupling effectively.
Data Coupling Example-4:
The Terrain Awareness and Warning System (TAWS) in an aircraft is designed to prevent Controlled Flight Into Terrain (CFIT). It does this by providing the flight crew with early warnings of a potential collision with terrain or obstacles.
For the sake of this example, let’s consider two classes – TerrainSensor and WarningSystem. TerrainSensor is responsible for gathering terrain data and WarningSystem uses this data to alert the crew of potential risks.
Here’s a basic example in C++:
// Module 1: Terrain Sensor class TerrainSensor { public: struct TerrainData { double altitude; double distance_to_ground; double upcoming_terrain_elevation; }; TerrainData getTerrainData() { TerrainData data; // Assume this function collects data from various terrain sensors data.altitude = /* Collected Altitude */; data.distance_to_ground = /* Collected Distance to Ground */; data.upcoming_terrain_elevation = /* Collected Upcoming Terrain Elevation */; return data; } };
// Module 2: Warning System class WarningSystem { public: void generateWarnings(TerrainSensor::TerrainData terrainData) { if (terrainData.altitude < 1000.0 && terrainData.distance_to_ground < 500.0) { // Code to generate "TOO LOW TERRAIN" warning } if (terrainData.upcoming_terrain_elevation > terrainData.altitude) { // Code to generate "TERRAIN AHEAD PULL UP" warning } } }; // Main function demonstrating data coupling int main() { TerrainSensor terrainSensor; WarningSystem warningSystem; TerrainSensor::TerrainData terrainData = terrainSensor.getTerrainData(); warningSystem.generateWarnings(terrainData); return 0; }
In this example, the TerrainSensor class collects data from various terrain sensors and returns a TerrainData struct. The WarningSystem class then reads the data from the TerrainData struct to generate the appropriate warnings. These classes are data coupled because the WarningSystem class directly depends on the data from the TerrainSensor class.
This is an example of simple data coupling, which is a low level of coupling and generally desirable. However, changes to the TerrainData struct (like renaming or removing any field) could potentially affect the WarningSystem class, emphasizing the importance of managing data coupling effectively.
Data Coupling Example-5:
In the context of aerospace software, let’s consider an example involving the Flight Management System (FMS). The FMS is a fundamental part of a modern aircraft’s avionics, and it relies heavily on the interaction between different systems, such as the navigation system and the autopilot system.
In Ada, we might have one package responsible for handling navigation data and another package that uses this navigation data to control the flight. Below is a simple demonstration of data coupling in this scenario:
-- Module 1: Navigation System package Navigation is type NavigationData is record Altitude : Float; Longitude : Float; Latitude : Float; end record; function GetNavigationData return NavigationData; end Navigation; package body Navigation is function GetNavigationData return NavigationData is Nav_Data : NavigationData; begin -- Assume this function collects data from various navigation sensors Nav_Data.Altitude := /* Collected Altitude */; Nav_Data.Longitude := /* Collected Longitude */; Nav_Data.Latitude := /* Collected Latitude */; return Nav_Data; end GetNavigationData; end Navigation;
-- Module 2: Flight Management System package FMS is procedure ControlFlight(Nav_Data : Navigation.NavigationData); end FMS; package body FMS is procedure ControlFlight(Nav_Data : Navigation.NavigationData) is begin if Nav_Data.Altitude < 1000.0 then -- Code to increase altitude elsif Nav_Data.Altitude > 5000.0 then -- Code to decrease altitude else -- Code to maintain current altitude end if; end ControlFlight; end FMS;
-- Main program demonstrating data coupling with Navigation; use Navigation; with FMS; use FMS; procedure Main is Nav_Data : NavigationData; begin Nav_Data := GetNavigationData; ControlFlight(Nav_Data); end Main;
In this example, the Navigation package collects data from various sensors and returns a NavigationData record. The FMS package then reads this data to control the flight. These modules are data coupled because the FMS package directly depends on the data from the Navigation package.
This is an example of simple data coupling, which is a low level of coupling and generally desirable. However, changes to the NavigationData record (like renaming or removing any field) could potentially affect the FMS package, emphasizing the importance of managing data coupling effectively.
The implication of data coupling on software maintainability and reliability:
Well-managed data coupling can contribute to software that is easier to maintain and more reliable. When modules are loosely coupled, changes can often be made to one without affecting others, which simplifies maintenance and testing. On the other hand, excessive or poorly managed data coupling can lead to software that is hard to maintain and prone to errors, as changes in one module could have unforeseen effects on others. In the critical context of aerospace software, this could potentially lead to safety issues.
Challenges of Data Coupling in Aerospace Software
Potential issues arising from data coupling:
While data coupling is essential for integrated system functionality, it can also introduce challenges. If data coupling is not carefully managed, it can lead to tightly coupled systems where changes in one module may have unforeseen effects on others. This can create maintenance difficulties, as modifications or bug fixes in one part of the software may cause problems in another. Additionally, extensive data coupling can result in more complex code, making it harder to understand, test, and debug.
Case studies of aerospace accidents linked to data coupling issues:
There have been incidents in the aerospace industry where poor data coupling management contributed to system failures. For instance, the 1996 Ariane 5 rocket explosion was partly due to a data conversion error between two software modules. The inertial reference system software (used in the previous Ariane 4) tried to pass a 64-bit floating-point number to the flight control system, which was expecting a 16-bit integer. This case underscores the potentially disastrous consequences of data coupling issues.
Impact of data coupling on the complexity of aerospace software:
Data coupling can significantly contribute to the complexity of aerospace software. When there’s a high degree of data coupling, it becomes challenging to comprehend the software system as a whole, as changes in one module can ripple through to affect many others. This complexity can make the software difficult to manage, update, and debug, which are critical tasks in the rapidly evolving aerospace industry. Furthermore, this complexity can also increase the risk of errors, which in the context of aerospace software, could have severe consequences.
Strategies to Manage Data Coupling in Aerospace Software
Best practices for managing data coupling in software development:
To manage data coupling effectively, software developers can follow several best practices. These include designing modules with clear and minimal interfaces, only sharing necessary data between modules, and using data abstraction to hide the internal details of data structures. Additionally, automated testing tools can help identify and mitigate potential data coupling issues by checking how changes in one module affect others.
Software design principles to reduce the impact of data coupling:
Several software design principles can help reduce the impact of data coupling. Principles like modularity, encapsulation, and information hiding are all aimed at minimizing the interdependencies between different parts of a software system. Using these principles, developers can design software where modules are largely independent and communicate through well-defined interfaces, reducing the impact of data coupling.
Application of these strategies in the aerospace sector:
In the aerospace sector, these strategies are particularly crucial given the high stakes involved. For example, using abstract data types or object-oriented programming can allow developers to encapsulate data within modules, reducing the chance of unexpected side effects from data coupling. Automated testing, including unit tests and integration tests, can help ensure that changes to one part of the aerospace software do not negatively affect other parts. Also, applying formal methods in the design process can help to ensure correctness and reliability despite data coupling.
Future Trends: Data Coupling and the Evolution of Aerospace Software
The impact of emerging technologies on data coupling:
Emerging technologies like Artificial Intelligence (AI) and Machine Learning (ML) are poised to have a significant impact on the management of data coupling in aerospace software. These technologies can potentially optimize the process of detecting and managing data dependencies, making software systems more robust and easier to maintain. Furthermore, the rise of distributed systems in aerospace, like swarm technologies in satellite systems, will further push the boundaries of managing data coupling.
Future challenges and opportunities for managing data coupling:
As aerospace systems become more complex and interconnected, managing data coupling will become an increasingly significant challenge. However, this also presents opportunities for the development of more advanced tools and methodologies for handling data coupling. Future aerospace systems will likely require new approaches to data coupling that can handle the increased complexity and interconnectivity of these systems.
How evolving aerospace technologies might shape data coupling practices:
The evolution of aerospace technologies will inevitably shape data coupling practices. For example, the trend towards more autonomous systems will require highly reliable software with well-managed data coupling to ensure system-wide integrity. The increased use of distributed systems and onboard processing capabilities in satellites and unmanned vehicles may also require new approaches to data coupling. The ongoing evolution of software development practices, including the adoption of agile methodologies and DevOps practices, will also likely influence data coupling management in aerospace software.
Conclusion
Summary of key points discussed:
Throughout this blog post, we have explored the concept of data coupling and its particular importance in aerospace software. We’ve delved into the various types of data coupling and examined the challenges and implications it poses in the development and maintenance of aerospace software systems. Furthermore, we have discussed several strategies for managing data coupling effectively and looked at how emerging technologies are shaping the future of data coupling in this field.
Reiteration of the importance of understanding and managing data coupling in aerospace software:
Understanding and managing data coupling is of utmost importance in aerospace software development due to the high-stakes, mission-critical nature of these systems. Well-managed data coupling can result in robust, maintainable, and reliable software. Conversely, poor data coupling can lead to software that is hard to maintain, prone to errors, and potentially dangerous. Therefore, it is essential for software engineers in the aerospace industry to be cognizant of data coupling and adopt best practices to manage it effectively.
Final thoughts and call to action for more research and awareness in this area:
As aerospace software systems continue to evolve in complexity and capability, the importance of managing data coupling effectively will only increase. There is a need for continued research and awareness in this area to develop advanced tools, techniques, and best practices that can handle the growing demands of aerospace software systems. By doing so, we can contribute to the creation of safer, more reliable, and more efficient aerospace systems for the future.
This post was published by Admin.
Email: admin@TheCloudStrap.Com